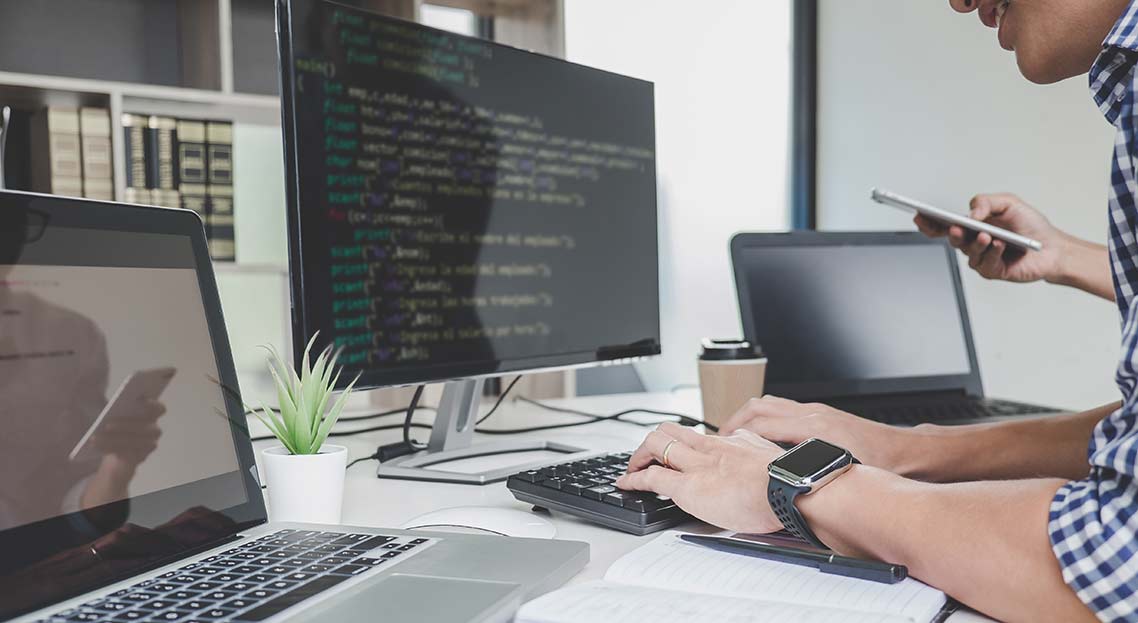
How to Use PowerShell Functions to Improve Readability of Your Scripts
When programming scripts to automate tasks, one of the recommended practices is creating functions to optimize the reusability of code. Administrators who automate multiple tasks with Microsoft Windows PowerShell will see that code lines are often repeated or need to separate large sections of code into different blocks to make scripts understandable and reduce coding time for writing additional scripts. PowerShell functions can solve this.
A function is a group of PowerShell statements. Like cmdlets, functions work with input parameters and can return values that can be displayed, assigned to variables, or piped to other functions or cmdlets. Once the function has been created, it may be called as many times as required instead of repeatedly repeating its code. There are two different types of functions in PowerShell: basic and advanced.
PowerShell Functions and Cmdlet Parameters
A cmdlet is a lightweight script you can use in a PowerShell environment to perform a single task such as changing directories or moving files. You can create cmdlets as PowerShell functions without writing and compiling a binary cmdlet. Hence, unlike functions that are integrated PowerShell environment before compiling, cmdlets are .NET classes that are already compiled into “.dll” files so that PowerShell can access them.
Like functions, cmdlets can have parameters and return values to display or assign to variables. You can add parameters to the cmdlet at the command prompt or pass them to other cmdlets via a pipeline as an output from the previous cmdlet. In this case, each parameter or argument’s values indicate the actual input that cmdlet accepts and how the cmdlets process such an input.
You can also incorporate switches (specialized arguments) that provide selections or presets for the parameter. For example, “Get-Help” is a simple cmdlet that you can use to get desired help concerning various commands. The syntax of getting support for a desired cmdlet from online documentation is:
Get-Help <cmd-name> -online
In the syntax above, the parameter<cmd-name> argument is the name of the command that you want to get more information about. For example, if you wanted to get more details about the Get-EventLog cmdlet with the switch “-online,” the PowerShell command would appear like this one:
Get-Help Get-EventLog -online
Add Parameters to your PowerShell Functions
You can also add parameters to the advanced functions that you write. These parameters are made available to your users in addition to the common parameters added by PowerShell to all cmdlets. Compiled cmdlets can carry out the culture-sensitive conversion, allows you to declare static parameters, and declare the attributes of parameters such as:
- Parameter Attribute: used to declare the attributes of function parameters.
- Mandatory Argument: used to declare a required parameter.
- Position Argument: used to determine if a parameter name is required when used in a command.
ParameterSetName argument, ValueFromPipeline Argument, ValueFromPipelineByPropertyName argument, HelpMessage Argument, Alias Attribute, SupportsWildcards Attribute etc.
Best Practices
To allow for consistent user experience with other cmdlets, adhere to the following best practices:
- Use a specific noun when naming cmdlet. The noun you choose should be specific and singular. For example, use “Get-Event” instead of “Get-Events.”
- Use the Pascal case when naming cmdlets. Always capitalize the first letter of the cmdlet verb and all noun terms. For example, use Get-Process instead of “Get-process.”
- Always use the standard parameter names. Using standard parameter names allows users to determine what the parameters entail quickly. For example, you should use “Get-Service” instead of “ServiceName.”
- Use consistent parameter types. When using the same parameter multiple cmdlets, ensure it is of the type.
Basic PowerShell Functions
Using Basic functions is the simplest option for administrators when working with PowerShell functions. These functions do not offer any built-in inherited features; hence, all types of error streams or parameter management requirements must be explicitly defined and covered in the function’s code.
The following image shows a basic function definition and its execution. This function lists all running services containing the string “RAS” in their Display Name:
Functions do not need to be complex to be helpful. Basic functions are often used to run simple tasks that do not require a complex parameter list or deep analysis on failed executions but are significantly important for a company’s day-to-day maintenance tasks.
Advanced PowerShell Functions
It’s really easy to convert a PowerShell function into an advanced function. One of the distinctions in between a regular function and an advanced form is that the latter includes a number of automated additions to the function of common parameters. These common settings include Verbose and Debug parameters.
Basic functions can be converted into advanced functions by simply adding the CmdletBinding attribute, the Parameter attribute, or both. The CmdletBinding attribute allows functions to operate like compiled cmdlets, thus enabling some of the built-in features that cmdlets provide, such as error streams. On the other hand, the Parameter attribute is used to predefine certain conditions of the function’s parameters such as establishing mandatory parameters.
Following the previous example, the function has been modified by adding the CmdletBinding attribute. Now, the PowerShell ISE auto-completion feature shows a list of built-in parameters that can be used to enhance the function performance.
Using advanced functions is a recommended practice when building scripts to be executed along separate environments and by different administrators because script programmers like common cmdlets can use them.
Parallels RAS PowerShell SDK
An SDK (Software Development Kit) is a collection of software tools and programs used by developers to manage specific products. SDK tools include some elements, libraries, processes, or documentation, and they are designed to be used with particular platforms and programming languages.
- Parallels® Remote Application Server (RAS) PowerShell SDK enables administrators to manage Parallels RAS Farms using Microsoft PowerShell scripting.
- It allows environment management and provisioning automation directly from the command line, in both on-premises and cloud scenarios, which increases compatibility with Microsoft Azure Cloud or Amazon Web Services.
- Parallels RAS PowerShell SDK can be installed as a standalone component, allowing administrators to manage and configure their RAS Farms from any location without interaction with the Parallels RAS Console.
- By combining RAS cmdlets and creating custom PowerShell functions, administrators will be able to build different scripts to manage and configure their RAS Farms.
- All common tasks can be easily automated with RAS cmdlets, from adding new users or managing sessions to creating newly published applications or the deployment of new desktops.
Check out the complete set of commands to manage a Parallels RAS Farm.